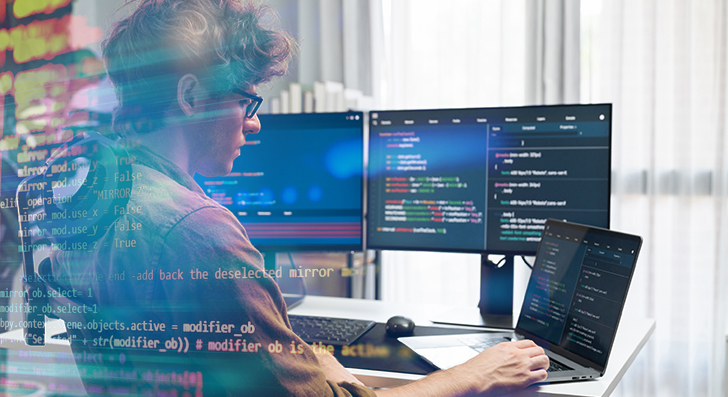
Scalability implies your software can cope with progress—more buyers, additional info, and even more visitors—with out breaking. As a developer, making with scalability in mind saves time and stress later on. Right here’s a transparent and useful guide to assist you to start off by Gustavo Woltmann.
Style for Scalability from the Start
Scalability just isn't some thing you bolt on afterwards—it should be aspect of one's approach from the beginning. Lots of programs fail if they develop speedy since the first style can’t cope with the extra load. Being a developer, you should Imagine early about how your technique will behave stressed.
Commence by designing your architecture for being adaptable. Avoid monolithic codebases the place every little thing is tightly linked. Instead, use modular design and style or microservices. These designs crack your app into smaller sized, impartial pieces. Every module or company can scale on its own with out impacting The full procedure.
Also, consider your database from day just one. Will it require to deal with 1,000,000 people or just a hundred? Choose the proper variety—relational or NoSQL—based upon how your details will increase. System for sharding, indexing, and backups early, Even when you don’t have to have them yet.
An additional critical position is to stop hardcoding assumptions. Don’t generate code that only is effective below existing problems. Think of what would transpire If the consumer foundation doubled tomorrow. Would your app crash? Would the database slow down?
Use design designs that help scaling, like message queues or event-driven units. These assistance your application cope with additional requests with no having overloaded.
After you Make with scalability in your mind, you're not just preparing for fulfillment—you might be cutting down foreseeable future head aches. A properly-planned system is less complicated to keep up, adapt, and expand. It’s far better to prepare early than to rebuild later.
Use the ideal Databases
Deciding on the suitable database is a vital Component of constructing scalable programs. Not all databases are built a similar, and utilizing the Incorrect one can gradual you down or maybe lead to failures as your app grows.
Get started by comprehension your info. Is it remarkably structured, like rows in the table? If Sure, a relational databases like PostgreSQL or MySQL is an efficient match. These are sturdy with relationships, transactions, and regularity. They also guidance scaling strategies like browse replicas, indexing, and partitioning to take care of a lot more traffic and knowledge.
If your details is much more adaptable—like consumer exercise logs, item catalogs, or paperwork—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling massive volumes of unstructured or semi-structured details and may scale horizontally additional easily.
Also, take into account your browse and create styles. Have you been executing lots of reads with less writes? Use caching and browse replicas. Are you handling a weighty generate load? Look into databases that will handle large publish throughput, or simply event-primarily based knowledge storage devices like Apache Kafka (for temporary information streams).
It’s also wise to Consider in advance. You may not want Innovative scaling options now, but choosing a database that supports them means you won’t require to switch later.
Use indexing to speed up queries. Stay away from avoidable joins. Normalize or denormalize your data depending on your access patterns. And always keep track of database overall performance as you grow.
In short, the proper database is dependent upon your app’s construction, speed needs, And exactly how you hope it to mature. Choose time to select correctly—it’ll preserve many difficulties later on.
Optimize Code and Queries
Fast code is essential to scalability. As your application grows, every single tiny delay provides up. Inadequately prepared code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s crucial to Develop efficient logic from the beginning.
Commence by creating clean, uncomplicated code. Keep away from repeating logic and remove anything pointless. Don’t pick the most intricate Answer if a straightforward one particular functions. Keep the features short, centered, and easy to check. Use profiling equipment to seek out bottlenecks—locations where by your code normally takes too very long to run or takes advantage of excessive memory.
Next, have a look at your database queries. These typically slow matters down a lot more than the code itself. Be sure each query only asks for the info you actually will need. Steer clear of Pick out *, which fetches every thing, and as a substitute select unique fields. Use indexes to speed up lookups. And prevent performing a lot of joins, Primarily across massive tables.
If you recognize the exact same data currently being asked for again and again, use caching. Retail outlet the results temporarily employing applications like Redis or Memcached so you don’t must repeat high priced functions.
Also, batch your databases operations whenever you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and helps make your application additional economical.
Make sure to test with huge datasets. Code and queries that operate high-quality with a hundred documents might crash once they have to deal with 1 million.
Briefly, scalable applications are rapidly applications. Maintain your code restricted, your queries lean, and use caching when essential. These techniques enable your software continue to be sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to handle much more consumers and a lot more targeted traffic. If almost everything goes by just one server, it is going to speedily become a bottleneck. That’s in which load balancing and caching are available in. These two tools aid keep your application speedy, steady, and scalable.
Load balancing spreads incoming targeted traffic across numerous servers. Rather than one server accomplishing many of the get the job done, the load balancer routes end users to distinct servers according to availability. This suggests no single server receives overloaded. If one particular server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this very easy to create.
Caching is about storing information quickly so it may be reused quickly. When buyers ask for precisely the same info all over again—like a product page or maybe a profile—you don’t must fetch it from the databases each time. You could serve it from the cache.
There are 2 common sorts of caching:
one. Server-facet caching (like Redis or Memcached) merchants information in memory for speedy accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) shops static data files close to the consumer.
Caching reduces database load, increases speed, and would make your app extra effective.
Use caching for things which don’t change typically. And always ensure your cache is current when information does transform.
In short, load balancing and caching are basic but powerful resources. Jointly, they assist your app handle a lot more people, stay quickly, and Get well from problems. If you plan to increase, you would like each.
Use Cloud and Container Equipment
To develop scalable purposes, you'll need equipment that allow your application develop simply. That’s wherever cloud platforms and containers are available. They give you flexibility, minimize setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really need to obtain components or guess future capacity. When site visitors will increase, it is possible to insert additional methods with just a couple clicks or mechanically working with car-scaling. When website traffic drops, you may scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and protection equipment. You may center on constructing your app as opposed to handling infrastructure.
Containers are another key Software. A container offers your app and every thing it needs to operate—code, libraries, options—into a single unit. This can make it effortless to move your application between environments, from a laptop computer towards the cloud, without surprises. Docker is the preferred Device for more info this.
When your application employs numerous containers, tools like Kubernetes make it easier to deal with them. Kubernetes handles deployment, scaling, and recovery. If just one element of your application crashes, it restarts it instantly.
Containers also make it easy to individual parts of your application into providers. You could update or scale areas independently, that is perfect for overall performance and trustworthiness.
In brief, applying cloud and container equipment means it is possible to scale fast, deploy simply, and recover speedily when problems come about. If you'd like your application to expand without the need of limits, start off using these equipment early. They help you save time, decrease possibility, and help you remain centered on building, not fixing.
Keep an eye on Everything
Should you don’t watch your software, you won’t know when items go Erroneous. Monitoring will help the thing is how your application is carrying out, place troubles early, and make improved decisions as your app grows. It’s a crucial Section of setting up scalable systems.
Commence by tracking standard metrics like CPU use, memory, disk House, and reaction time. These show you how your servers and services are performing. Equipment like Prometheus, Grafana, Datadog, or New Relic will let you collect and visualize this information.
Don’t just check your servers—keep an eye on your app way too. Control just how long it will require for people to load web pages, how frequently glitches transpire, and wherever they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s occurring within your code.
Put in place alerts for critical troubles. By way of example, When your reaction time goes earlier mentioned a limit or even a services goes down, you need to get notified instantly. This helps you fix challenges rapid, typically ahead of consumers even discover.
Checking is likewise valuable once you make modifications. If you deploy a completely new attribute and see a spike in faults or slowdowns, you may roll it back again before it leads to serious destruction.
As your app grows, visitors and details enhance. With out checking, you’ll overlook signs of difficulties until finally it’s much too late. But with the best resources set up, you remain on top of things.
In brief, checking aids you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about knowing your procedure and ensuring it works very well, even under pressure.
Closing Thoughts
Scalability isn’t just for significant organizations. Even compact apps will need a strong Basis. By building very carefully, optimizing sensibly, and using the appropriate applications, you'll be able to Establish apps that increase effortlessly with out breaking stressed. Get started little, Assume big, and Construct clever.